The most important component of the Spring Framework is the Spring Core Container which creates the objects, wires them together, manages the configuration and overall object life cycle from creation of the object till its destruction. These objects are called Beans which are created from the configuration metadata provided to the container. Configuration Metadata provides the information to the container with regard to bean
- Managing Bean Creation Process
2. Managing life cycle of the bean
3. Managing dependencies of the bean
Configuration Metadata Properties
Below are the commonly used properties for the configuration metadata in spring bean scope.
Name – specifies bean identifier
Class – specifies the bean class for which bean is to be created
Constructor –arg – specifies the dependency injection
Scope – specifies the scope of the object created from the bean definition
Properties – specifies properties for the dependency injection
Auto wiring Mode – specifies the dependency injection
lazy-initialization mode – specifies the lazy initiation of the bean instance. That is, bean instance is created when it is requested and not at the start-up of the application
initialization method – A callback to be called just after all necessary properties on the bean have been set by the container
destruction method – A callback to be used when the container containing the bean is destroyed
Configuration Metadata Methodologies
Configuration Metadata can be provided by using the below given methodologies
- XML based configuration file.
- Annotation-based configuration
- Java-based configuration
Sample XML Configuration
<?xml version = "1.0" encoding = "UTF-8"?>
<beans xmlns = "http://www.springframework.org/schema/beans"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<!-- A simple bean definition -->
<bean id = "..." class = "createCustomer">
</bean>
<!-- A bean definition with lazy init set on -->
<bean id = "..." class = "..." lazy-init = "true">
</bean>
<!-- A bean definition with initialization method -->
<bean id = "..." class = "..." init-method = "Initiate-customer-process">
</bean>
<!-- A bean definition with destruction method -->
<bean id = "..." class = "..." destroy-method = "destruct-customer-process">
</bean>
</beans>
Spring supported Bean Scope
The Spring Framework supports the below given bean scopes.
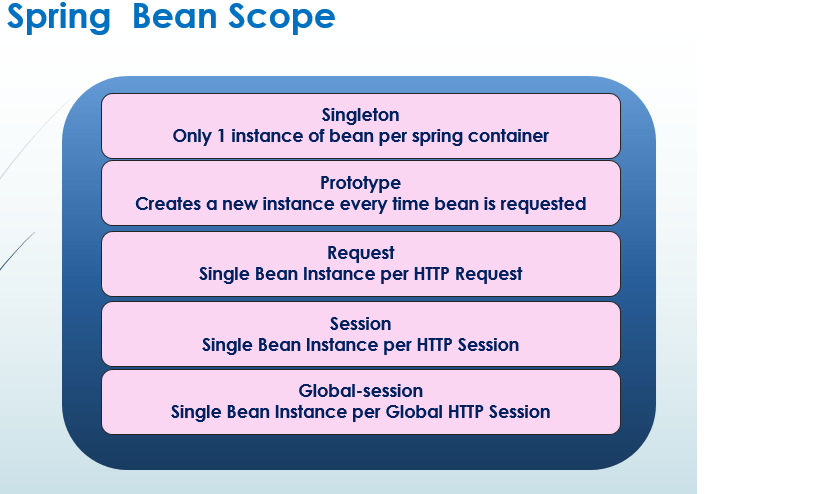
Singleton Scope: With the use of singleton scope, Spring IOC Container creates only 1 bean instance of the object defined by the bean definition.
The below bean configuration file does not include the scope specified and thus makes it as a singleton instance.
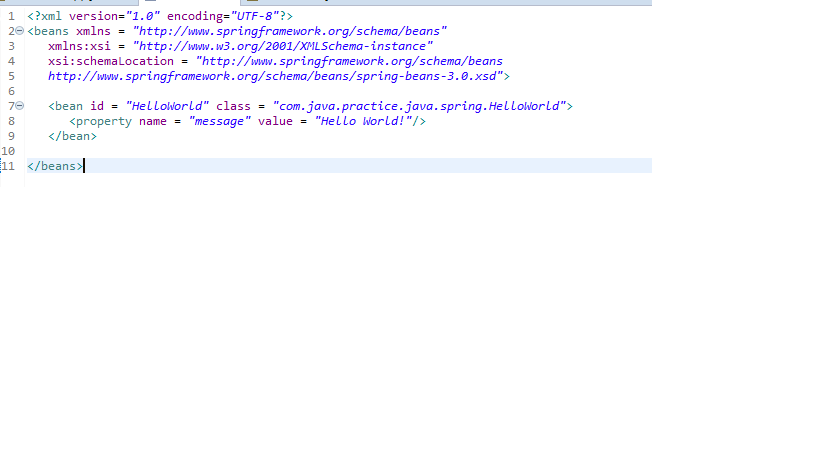
Another bean configuration with the usage of scope as “singleton”
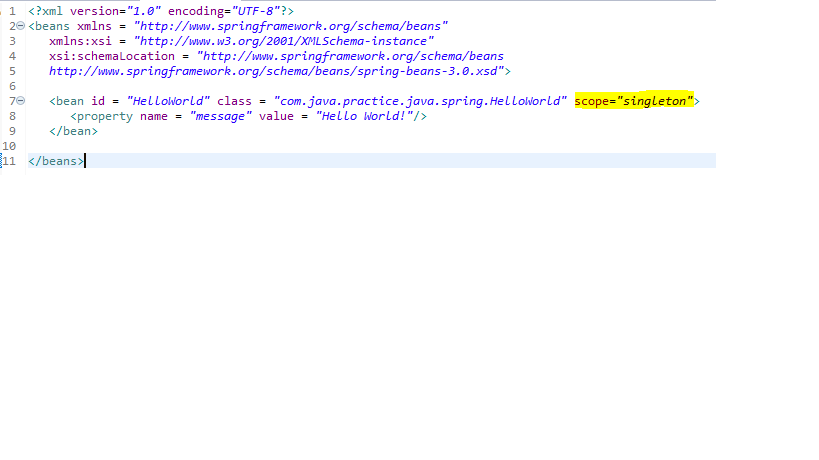
Prototype Bean Scope
With the use of prototype scope, Spring IOC Container creates a new bean instance every time the bean is requested.
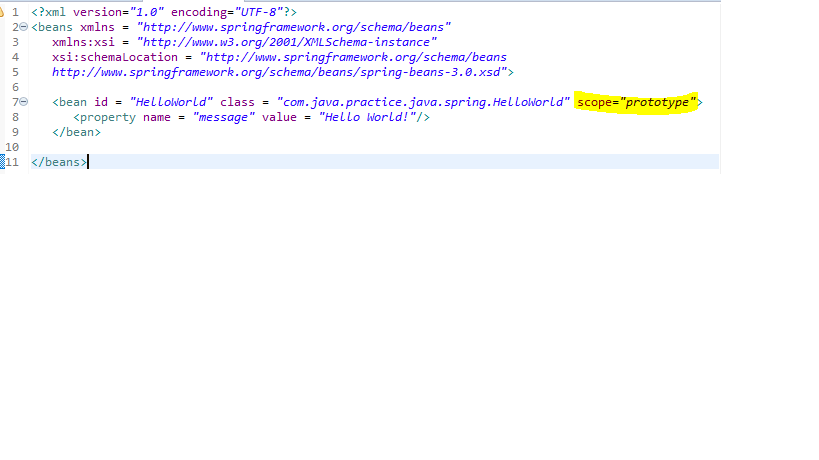
Bean Scope Annotation
Spring also provides annotation to define the bean scope as an alternative to the XML based configuration. The annotation based bean depends on bytecode metadata for wiring up components instead of angle-bracket declarations and allows developers to write the configuration into the component class itself by using annotations on the relevant class, method, or field declaration.
The RequiredAnnotationBeanPostProcessor, using a BeanPostProcessor in conjunction with annotations is a common means of extending the Spring IOC container.
Spring 2.5 also added support for JSR-250 annotations, such as @PostConstruct and @PreDestroy.
Spring 3.0 added support for JSR-330 (Dependency Injection for Java) annotations contained in the javax.inject package such as @Inject and @Named
