The tutorial provides the details about the Node.js Cypto module . The crypto module includes the set of wrappers for open SSL’s hash HMAC, cipher, decipher, sign and verify functions for providing cryptographic functionality. We need to include require(‘crypto’) as the module . Let’s see the simple example .
Example to know if crypto module is enabled or not
let crypto; try { crypto = require('crypto'); console.log('Crypto is enabled'); } catch (err) { console.log('crypto support is disabled!'); }

What is Hash in Node.js crypto module ?
A hash is a fixed-length string of bits generated from some arbitrary block of source data.
What is HMAC in Node.js crypto module ?
HMAC means Hash-based Message Authentication Code.
HMAC is the process for applying a hash algorithm to both data and a secret key that results in a single final hash.
node.js crypto example using Hash and HMAC
const crypto = require('crypto'); const secret = '122112'; const hash = crypto.createHmac('sha256', secret) .update('This is node.js crypto example') .digest('hex'); console.log(hash);

Node.js Class Certificate in crypto module
The node.js crypto module uses the SPKAC ( Certificate Signing Request mechanism originally implemented by Netscape ) for providing the certificate. OpenSSLs SPKAC Implementation details can be found here- https://www.openssl.org/docs/man1.1.0/man1/openssl-spkac.html
Node.js Class Cipher in crypto module
The node.js uses the class Cipher for encrypting the data in the below given ways:
- As part of streaming , plain unencrypted data to convert into encrypted data form that is both readable and writeable
- impementing the cipher.update() and cipher.final() method for encrypting the data.
The cipher objects cannot be created using the new Keyword. For creating the cipher instances, the crypto.createCipher() or crypto.createCipheriv() methods can be used
const crypto = require('crypto'); const cipher = crypto.createCipher('mks212', 'password'); var convert_to_encrypted_data = cipher.update('Using Cipher for encryption in crypto module', 'utf8', 'hex'); convert_to_encrypted_data += cipher.final('hex'); console.log(convert_to_encrypted_data);

Run time example in case of error for Cipher creation
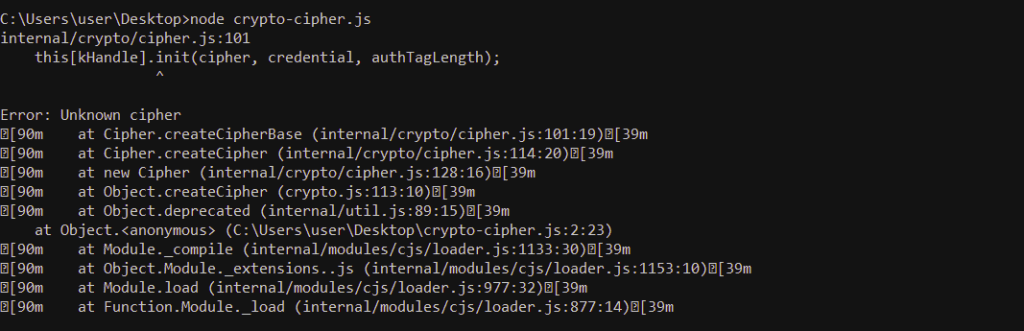
Node.js Class Decipher in crypto module
The node.js uses the class Decipher for decrypting the data in the below given ways:
- As part of streaming , plain encrypted data to convert into decrypted data form that is both readable and writeable
- impementing the decipher.update() and decipher.final() method for decrypting the data.
The decipher objects cannot be created using the new Keyword. For creating the decipher instances, the crypto.createDecipher() or crypto.createDecipheriv() methods can be used
const crypto = require('crypto'); const decipher = crypto.createDecipher('mks212', 'password'); var encrypted = 'c414206f46af864a92b11c4f1273cd87718624840cb72956c48f60df8b12882f898c185115598b8a16a80629ef41aedb'; var convertodecrypteddata = decipher.update(encrypted, 'hex', 'utf8'); convertodecrypteddata += decipher.final('utf8'); console.log(convertodecrypteddata);